The script that was posted here, didn’t give me the result I wanted (it didn’t give me a result at all).
It took me a while to get things working for me, as I’m no expert in scripting.
This script runs between sunrise – 30 min and sunset +30 min
After that it looks at the state of the converter, if it is running than it will retrieve data and update the sensors.
Code: Select all
-- Script to read solardata from FroniusAPI
-- Autor : Meulderg
-- Date : 29-Jan-2017
-- Name: script_time_Fronius.lua
-- Warning: Don't forget to setup the Local Networks otherwize the device counters won't get updated if site security is used.
-- Variables to customize ------------------------------------------------
local DEBUG = 0 -- 0 is off, 1 for domoticz log, 2 for fronius JSON data to log
local IPdomiticz = '192.168.xxx.xxx:8080' -- IP adress of the domoticz service + port
local IPFronius = '192.168.xxx.xxx' -- IP adress of the Fronius converter
local idx_Fronius_Opbrengst = 19 -- idx of the virtual sensor, you need to change this to your own Device IDx
local idx_UDC = 16 -- idx of the virtual sensor, you need to change this to your own Device IDx
local idx_UAC = 15 -- idx of the virtual sensor, you need to change this to your own Device IDx
local idx_IDC = 18 -- idx of the virtual sensor, you need to change this to your own Device IDx
local idx_IAC = 17 -- idx of the virtual sensor, you need to change this to your own Device IDx
local idx_DAY_ENERGY = 12 -- idx of the virtual sensor, you need to change this to your own Device IDx
local idx_YEAR_ENERGY = 13 -- idx of the virtual sensor, you need to change this to your own Device IDx
local idx_TOTAL_ENERGY = 14 -- idx of the virtual sensor, you need to change this to your own Device IDx
--Create Virtual Hardware via JSON script (Copy this URL manualy)
--Change IP, Port and name accordingly
--http://127.0.0.1:8080/json.htm?type=command¶m=addhardware&htype=15&port=1&name=Fronius&enabled=true
--Create Virtual sensors via JSON script (Copy these URL manual)
--Change IP, Port and idx (idx is of the previously created hardware ID) accordingly
--http://127.0.0.1:8080/json.htm?type=createvirtualsensor&idx=4&sensorname=Fronius_Opbrengst&sensortype=18
--Above sensor needs to be manual adjusted to Type: Return.
--http://127.0.0.1:8080/json.htm?type=createvirtualsensor&idx=4&sensorname=Fronius_Day_Energy&sensortype=248
--http://127.0.0.1:8080/json.htm?type=createvirtualsensor&idx=4&sensorname=Fronius_Year_Energy&sensortype=248
--http://127.0.0.1:8080/json.htm?type=createvirtualsensor&idx=4&sensorname=Fronius_Total_Energy&sensortype=248
--http://127.0.0.1:8080/json.htm?type=createvirtualsensor&idx=4&sensorname=Fronius_UAC&sensortype=4
--http://127.0.0.1:8080/json.htm?type=createvirtualsensor&idx=4&sensorname=Fronius_UDC&sensortype=4
--http://127.0.0.1:8080/json.htm?type=createvirtualsensor&idx=4&sensorname=Fronius_IAC&sensortype=19
--http://127.0.0.1:8080/json.htm?type=createvirtualsensor&idx=4&sensorname=Fronius_IDC&sensortype=19
-- one-time load of the routines
JSON = (loadfile "C:\\Domoticz\\scripts\\lua\\json.lua")() -- For Windows
-- JSON = (loadfile "/home/pi/domoticz/scripts/lua/JSON.lua")() -- For Linux
commandArray = {}
if(DEBUG == 1) then print ('Fronius script running!!') end
-- get current time & calculate sunrise and sunset.
time_now = os.date("*t")
minutes_now = time_now.min + time_now.hour * 60
if(DEBUG == 1) then print ('Time in minutes: ' ..minutes_now) end
if(DEBUG == 1) then print ('Sunrise in minutes: ' ..timeofday['SunriseInMinutes'] - 60) end
if(DEBUG == 1) then print ('Sunset in minutes: ' ..timeofday['SunsetInMinutes'] + 60) end
-- Validation for sunrise and sunset.
if (minutes_now > timeofday['SunriseInMinutes'] - 30) and (minutes_now < timeofday['SunsetInMinutes'] + 30) then
--Extract data from Fronius converter.
froniusurl = 'curl "http://'..IPFronius..'/solar_api/v1/GetInverterRealtimeData.cgi?Scope=Device&DeviceID=1&DataCollection=CommonInverterData"'
jsondata = assert(io.popen(froniusurl))
froniusdevice = jsondata:read('*all')
jsondata:close()
froniusdata = JSON:decode(froniusdevice)
--Process fronius data
if (froniusdata ~= nil) then
if(DEBUG == 2) then print (froniusdevice) end
local StatusCode = froniusdata['Body']['Data']['DeviceStatus']['StatusCode']
if( StatusCode == 7) then --Fronius converter is Running
local DAY_ENERGY = froniusdata['Body']['Data']['DAY_ENERGY']['Value']
local YEAR_ENERGY = froniusdata['Body']['Data']['YEAR_ENERGY']['Value']
local TOTAL_ENERGY = froniusdata['Body']['Data']['TOTAL_ENERGY']['Value']
local PAC = froniusdata['Body']['Data']['PAC']['Value']
local UDC = froniusdata['Body']['Data']['UDC']['Value']
local UAC = froniusdata['Body']['Data']['UAC']['Value']
local IDC = froniusdata['Body']['Data']['IDC']['Value']
local IAC = froniusdata['Body']['Data']['IAC']['Value']
local UDC = froniusdata['Body']['Data']['UDC']['Value']
if(DEBUG == 1) then print ('PAC: '..PAC) end
if(DEBUG == 1) then print ('Day Energy: '..DAY_ENERGY) end
if(DEBUG == 1) then print ('Year Energy: '..YEAR_ENERGY) end
if(DEBUG == 1) then print ('Total Energy: '..TOTAL_ENERGY) end
if(DEBUG == 1) then print ('UDC: '..UDC) end
if(DEBUG == 1) then print ('UAC: '..UAC) end
if(DEBUG == 1) then print ('IDC: '..IDC) end
if(DEBUG == 1) then print ('IAC: '..IAC) end
--To update the devices.
commandArray[#commandArray + 1]={['OpenURL']="http://"..IPdomiticz.."/json.htm?type=command¶m=udevice&idx="..idx_Fronius_Opbrengst.."&nvalue=0&svalue="..PAC..";"..DAY_ENERGY..""}
commandArray[#commandArray + 1]={['OpenURL']="http://"..IPdomiticz.."/json.htm?type=command¶m=udevice&idx="..idx_UDC.."&nvalue=0&svalue="..UDC..""}
commandArray[#commandArray + 1]={['OpenURL']="http://"..IPdomiticz.."/json.htm?type=command¶m=udevice&idx="..idx_IDC.."&nvalue=0&svalue="..IDC..""}
commandArray[#commandArray + 1]={['OpenURL']="http://"..IPdomiticz.."/json.htm?type=command¶m=udevice&idx="..idx_UAC.."&nvalue=0&svalue="..UAC..""}
commandArray[#commandArray + 1]={['OpenURL']="http://"..IPdomiticz.."/json.htm?type=command¶m=udevice&idx="..idx_IAC.."&nvalue=0&svalue="..IAC..""}
commandArray[#commandArray + 1]={['OpenURL']="http://"..IPdomiticz.."/json.htm?type=command¶m=udevice&idx="..idx_DAY_ENERGY.."&nvalue=0&svalue="..DAY_ENERGY..""}
commandArray[#commandArray + 1]={['OpenURL']="http://"..IPdomiticz.."/json.htm?type=command¶m=udevice&idx="..idx_YEAR_ENERGY.."&nvalue=0&svalue="..YEAR_ENERGY..""}
commandArray[#commandArray + 1]={['OpenURL']="http://"..IPdomiticz.."/json.htm?type=command¶m=udevice&idx="..idx_TOTAL_ENERGY.."&nvalue=0&svalue="..TOTAL_ENERGY..""}
else
print ('Fronius converter state other than running')
local UDC = 0 local UAC = 0 local IDC = 0 local IAC = 0
commandArray[#commandArray + 1]={['OpenURL']="http://"..IPdomiticz.."/json.htm?type=command¶m=udevice&idx="..idx_UDC.."&nvalue=0&svalue="..UDC..""}
commandArray[#commandArray + 1]={['OpenURL']="http://"..IPdomiticz.."/json.htm?type=command¶m=udevice&idx="..idx_IDC.."&nvalue=0&svalue="..IDC..""}
commandArray[#commandArray + 1]={['OpenURL']="http://"..IPdomiticz.."/json.htm?type=command¶m=udevice&idx="..idx_UAC.."&nvalue=0&svalue="..UAC..""}
commandArray[#commandArray + 1]={['OpenURL']="http://"..IPdomiticz.."/json.htm?type=command¶m=udevice&idx="..idx_IAC.."&nvalue=0&svalue="..IAC..""}
end
else
print ('Fronius converter data is empty or unreachable')
end
else
print ('Fronius converter Offline')
end
return commandArray
-- Example of the data returned by the Fronius converter
--[[
{
"Head" : {
"RequestArguments" : {
"DataCollection" : "CommonInverterData",
"DeviceClass" : "Inverter",
"DeviceId" : "1",
"Scope" : "Device"
},
"Status" : {
"Code" : 0,
"Reason" : "",
"UserMessage" : ""
},
"Timestamp" : "2017-01-29T12:16:22+01:00"
},
"Body" : {
"Data" : {
"DAY_ENERGY" : {
"Value" : 1535.1,
"Unit" : "Wh"
},
"FAC" : {
"Value" : 50,
"Unit" : "Hz"
},
"IAC" : {
"Value" : 4.68,
"Unit" : "A"
},
"IDC" : {
"Value" : 3.49,
"Unit" : "A"
},
"PAC" : {
"Value" : 1062,
"Unit" : "W"
},
"TOTAL_ENERGY" : {
"Value" : 839677.06,
"Unit" : "Wh"
},
"UAC" : {
"Value" : 231.1,
"Unit" : "V"
},
"UDC" : {
"Value" : 342.7,
"Unit" : "V"
},
"YEAR_ENERGY" : {
"Value" : 119222.5,
"Unit" : "Wh"
},
"DeviceStatus" : {
"StatusCode" : 7,
"MgmtTimerRemainingTime" : -1,
"ErrorCode" : 0,
"LEDColor" : 2,
"LEDState" : 0,
"StateToReset" : false
}
}
}
}
]]--
This code is used on a windows based domoticz.
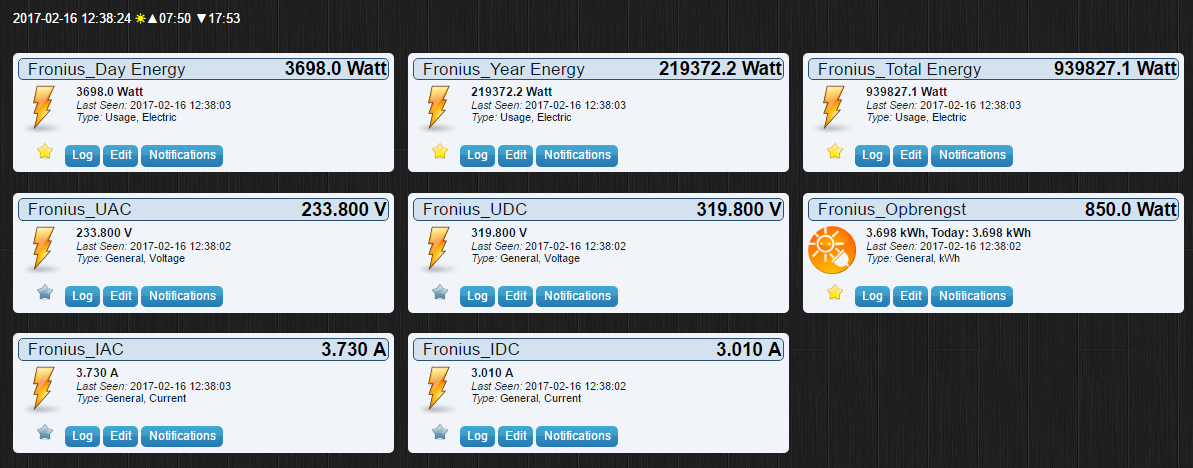
- Sensors Fronius
- 2017-02-16_Fronius.png (100.13 KiB) Viewed 7492 times