Latest Version of the code updated : 10/03/2015 V0.4 <- Latest version updated to support new temperature set point switch. this removes the need for lots of the code and additional switches. Enjoy

I thought I'd share as I finally managed to get my heating hooked up to Domoticz. I know some of you already have something like this but I'm well pleased now I have it working. See below a screenshot and code for anyone who wants it. Have fun.
Hardware:
Woostesher Bosch CDi 30 - Link: http://www.worcester-bosch.co.uk/homeow ... sic-system
Homeeasy 105 Switch (Currently on sale - http://www.domoticz.com/forum/viewtopic.php?f=18&t=4028)
rfxtrx & pi

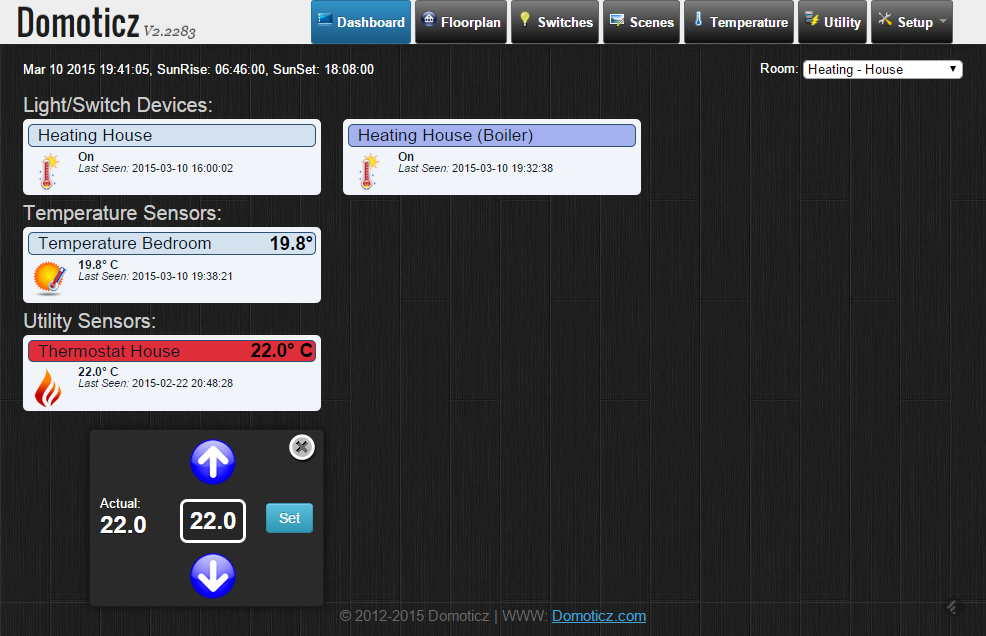
Latest Version of the code updated : 08/11/2015 V0.4.1
Code: Select all
-----------------------------------------------------------------------------------------------
-- Heating Control
-- Version 0.4.1
-- Author - Martin Rourke
-- This library is free software: you can redistribute it and/or modify it under the terms of
-- the GNU Lesser General Public License as published by the Free Software Foundation, either
-- version 3 of the License, or (at your option) any later version. This library is distributed
-- in the hope that it will be useful, but WITHOUT ANY WARRANTY; without even the implied
-- warranty of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General
-- Public License for more details. You should have received a copy of the GNU Lesser General
-- Public License along with this library. If not, see <http://www.gnu.org/licenses/>.
-----------------------------------------------------------------------------------------------
-----------------------------------------------------------------------------------------------
-- Setup
-----------------------------------------------------------------------------------------------
-- To use this script you need to have a few devices already setup in Domoticz
-- 1. A physical switch to operate (On/Off Switch)
-- 2. A physical temperature sensor (Temperature)
-- 3. A virtual switch to tun on and off the heating (On/Off Switch)
-- 4. A virtual switch to set the target temperature (Temperature)
-- 5. Define a User Variable as 'Debug', Integer and set to 1 or 0 to show/hide entries in the log
-----------------------------------------------------------------------------------------------
-- 1. A physical switch to operate (On/Off Switch)
-----------------------------------------------------------------------------------------------
local physicalheater = 'Heating House (Boiler)'
-----------------------------------------------------------------------------------------------
-- 2. A physical temperature sensor (Temperature)
-----------------------------------------------------------------------------------------------
-- Future improvement will be to define more than one temperature sensor and assume an average
-----------------------------------------------------------------------------------------------
local currenttemperature = 'Temperature Bedroom'
-----------------------------------------------------------------------------------------------
-- 3. A virtual switch to tun on and off the heating (On/Off Switch)
-----------------------------------------------------------------------------------------------
local virtualheater = 'Heating House'
-----------------------------------------------------------------------------------------------
-- 4. A virtual switch to set the target temperature (Temperature)
-- Assumes you are using a dummy switch "Thermostat Setpoint" to move temperature up and down
-----------------------------------------------------------------------------------------------
local targettemperature = 'Thermostat House'
-----------------------------------------------------------------------------------------------
-- 5. UserVariable - Set debug to 1 for testing. 0 for live running.
-----------------------------------------------------------------------------------------------
-----------------------------------------------------------------------------------------------
-- OPTIONS
-----------------------------------------------------------------------------------------------
-- Defines the amount the target temperature will move when the up and down buttons are pressed
-----------------------------------------------------------------------------------------------
local pitch= 1
-----------------------------------------------------------------------------------------------
-- The tolerance for the thermostat. So the heating doesn't continually turn on and off around
-- the target temperature this specifies the tolerance before any action is taken.
-----------------------------------------------------------------------------------------------
local tolerance = 0.5 -- NOT BEING USED!!!!!
-----------------------------------------------------------------------------------------------
-- After this time the heating will automatically turn off as a temperature was not detected
-- from the temperature sensor. this helps protect against the heating being left on all the
-- time if the signal is lost.
-----------------------------------------------------------------------------------------------
local lostsignaltime = 3600 -- 60 minutes
-----------------------------------------------------------------------------------------------
-- Helps protect against multiple quick changes to the physical switch
-----------------------------------------------------------------------------------------------
local heatercommanddelay = 30 -- 30 seconds
-----------------------------------------------------------------------------------------------
-- Repeats the On or Off command after a set time for devices that don't have a handshake
-- Should always be bigger than heatercommanddelay
-----------------------------------------------------------------------------------------------
local heaterrepeatdelay = 60 -- 1 minute
-----------------------------------------------------------------------------------------------
-----------------------------------------------------------------------------------------------
-----------------------------------------------------------------------------------------------
-----------------------------------------------------------------------------------------------
-----------------------------------------------------------------------------------------------
-- NOTHING SHOULD NEED TO CHANGE BELOW THIS LINE
-----------------------------------------------------------------------------------------------
-----------------------------------------------------------------------------------------------
-----------------------------------------------------------------------------------------------
-----------------------------------------------------------------------------------------------
-----------------------------------------------------------------------------------------------
local thermostatchgnagedetected = 0
commandArray = {}
function debuglog (s)
if uservariables["Debug"] == 1 then print("DEBUG - THERMOSTAT " .. physicalheater .. s) end
return true
end
function timedifference (s)
year = string.sub(s, 1, 4)
month = string.sub(s, 6, 7)
day = string.sub(s, 9, 10)
hour = string.sub(s, 12, 13)
minutes = string.sub(s, 15, 16)
seconds = string.sub(s, 18, 19)
t1 = os.time()
t2 = os.time{year=year, month=month, day=day, hour=hour, min=minutes, sec=seconds}
difference = os.difftime (t1, t2)
return difference
end
-----------------------------------------------------------------------------------------------
-- Controls the heating based on the target and current temperatures
-----------------------------------------------------------------------------------------------
-- Is the heating scheduled to be on?
if (otherdevices[virtualheater]=='On') then
debuglog('Heating - Heating Is Scheduled To Be On: ' .. virtualheater )
-- what is the target temperature?
target = tonumber(otherdevices_svalues[targettemperature])
-- test to see if we can still see the thermostat. If not force the heating off
--debuglog('Heating - Control Switch: ' .. tostring(timedifference(otherdevices_lastupdate[currenttemperature])) .. ' LostSignalTime: ' .. tostring(lostsignaltime))
if ( timedifference(otherdevices_lastupdate[currenttemperature]) > lostsignaltime ) then
debuglog('Heating - WARNING: Last seen temperature sensor: '.. currenttemperature ..' ' .. tostring(otherdevices_lastupdate[currenttemperature]))
debuglog('Heating - WARNING: Signal Lost with temperature sensor for over '.. lostsignaltime ..' seconds: '.. currenttemperature)
debuglog('Heating - WARNING: forcing Heating OFF : '.. physicalheater)
if( timedifference(otherdevices_lastupdate[physicalheater]) > heaterrepeatdelay) then -- checks to see if the command needs to be repeated
if (otherdevices[physicalheater]=='Off') then
debuglog('Heating - WARNING - Turning Physical Heater On: '.. physicalheater)
else
debuglog('Heating - WARNING - Turning Physical Heater On (Repeat Command): '.. physicalheater)
end
commandArray[physicalheater] = 'On'
else
debuglog('Heating - INFO: Not enough time has passed before issuing repeat command to switch: Only '.. timedifference(otherdevices_lastupdate[physicalheater]) .. ' seconds out of ' .. heaterrepeatdelay .. ' seconds')
end
else
debuglog('Heating - Last seen temperature sensor: '.. currenttemperature ..' ' .. tostring(otherdevices_lastupdate[currenttemperature]))
-- What is the current temperature?
--debuglog('STRING 1 Current - Return Value : ' .. tostring(otherdevices_svalues[currenttemperature]))
--debuglog('STRING 2 Current - Value Type : ' .. type(otherdevices_svalues[currenttemperature]))
--debuglog('STRING 3 Current - First Element : ' .. string.gmatch(otherdevices_svalues[currenttemperature], '([^;]+)')(1))
-- As some temperature devices also return other values as humidity this assumes the first value is the temperature in all cases
current = tonumber(string.gmatch(otherdevices_svalues[currenttemperature], '([^;]+)')(1))
debuglog('Heating - Target: ' .. tostring(target) .. ' Current: ' .. tostring(current))
if( timedifference(otherdevices_lastupdate[physicalheater]) > heatercommanddelay ) then -- checks to see if the heater has been turned off recently
debuglog('Heating - Enough time has passed since last change to switch: '.. timedifference(otherdevices_lastupdate[physicalheater]) .. ' seconds out of ' .. heatercommanddelay .. ' seconds')
if (current < target) then
debuglog('Heating - Turning Physical Heater On: '.. physicalheater)
if( timedifference(otherdevices_lastupdate[physicalheater]) > heaterrepeatdelay or devicechanged[remoteup] or devicechanged[remotedown] or devicechanged[virtualheater]) then -- checks to see if the command needs to be repeated
if (otherdevices[physicalheater]=='Off') then
debuglog('Heating - Turning Physical Heater On: '.. physicalheater)
else
debuglog('Heating - Turning Physical Heater On (Repeat Command): '.. physicalheater)
end
commandArray[physicalheater] = 'On'
else
debuglog('Heating - INFO: Not enough time has passed before issuing repeat command to switch: Only '.. timedifference(otherdevices_lastupdate[physicalheater]) .. ' seconds out of ' .. heaterrepeatdelay .. ' seconds')
end
else
debuglog('Heating - Turning Physical Heater Off: '.. physicalheater)
if( timedifference(otherdevices_lastupdate[physicalheater]) > heaterrepeatdelay or devicechanged[remoteup] or devicechanged[remotedown] or devicechanged[virtualheater]) then -- checks to see if the command needs to be repeated
if (otherdevices[physicalheater]=='On') then
debuglog('Heating - Turning Physical Heater Off: '.. physicalheater)
else
debuglog('Heating - Turning Physical Heater Off (Repeat Command): '.. physicalheater)
end
commandArray[physicalheater] = 'Off'
else
debuglog('Heating - INFO: Not enough time has passed before issuing repeat command to switch: Only '.. timedifference(otherdevices_lastupdate[physicalheater]) .. ' seconds out of ' .. heaterrepeatdelay .. ' seconds')
end
end
else
debuglog('Heating - INFO: Not enough time has passed before issuing command to switch: Only '.. timedifference(otherdevices_lastupdate[physicalheater]) .. ' seconds out of ' .. heatercommanddelay .. ' seconds')
end
end
else
debuglog('Heating - Turning Heating Off As Scheduled To Be Off')
if( timedifference(otherdevices_lastupdate[physicalheater]) > heatercommanddelay ) then -- NEED SOMETHING IN HERE TO STOP TURNING ON AND OFF CONSTANTLY
debuglog('Heating - Enough time has passed before issuing command to switch: '.. timedifference(otherdevices_lastupdate[physicalheater]) .. ' seconds out of ' .. heatercommanddelay .. ' seconds')
if( timedifference(otherdevices_lastupdate[physicalheater]) > heaterrepeatdelay or devicechanged[remoteup] or devicechanged[remotedown] or devicechanged[virtualheater]) then -- checks to see if the command needs to be repeated
if (otherdevices[physicalheater]=='On') then
debuglog('Heating - Turning Physical Heater Off: '.. physicalheater)
else
debuglog('Heating - Turning Physical Heater Off (Repeat Command): '.. physicalheater)
end
commandArray[physicalheater] = 'Off'
else
debuglog('Heating - INFO: Not enough time has passed before issuing repeat command to switch: Only '.. timedifference(otherdevices_lastupdate[physicalheater]) .. ' seconds out of ' .. heaterrepeatdelay .. ' seconds')
end
else
debuglog('Heating - INFO: Not enough time has passed before issuing command to switch: Only '.. timedifference(otherdevices_lastupdate[physicalheater]) .. ' seconds out of ' .. heatercommanddelay .. ' seconds')
end
end
Code: Select all
-----------------------------------------------------------------------------------------------
-- Heating Control
-- Version 0.3
-- Author - Martin Rourke
-- This library is free software: you can redistribute it and/or modify it under the terms of
-- the GNU Lesser General Public License as published by the Free Software Foundation, either
-- version 3 of the License, or (at your option) any later version. This library is distributed
-- in the hope that it will be useful, but WITHOUT ANY WARRANTY; without even the implied
-- warranty of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General
-- Public License for more details. You should have received a copy of the GNU Lesser General
-- Public License along with this library. If not, see <http://www.gnu.org/licenses/>.
-----------------------------------------------------------------------------------------------
-----------------------------------------------------------------------------------------------
-- Setup
-----------------------------------------------------------------------------------------------
-- To use this script you need to have a few devices already setup in Domoticz
-- 1. A physical switch to operate (On/Off Switch)
-- 2. A physical temperature sensor (Temperature)
-- 3. A virtual switch to tun on and off the heating (On/Off Switch)
-- 4. A virtual switch to set the target temperature (Temperature)
-- 5. A virtual switch to move the target temperature up (Push Button)
-- 6. A virtual switch to move the target temperature down (Push Button)
-----------------------------------------------------------------------------------------------
-- 1. A physical switch to operate (On/Off Switch)
-----------------------------------------------------------------------------------------------
local physicalheater = 'Heating House (Boiler)'
local physicalheateridx = 181
-----------------------------------------------------------------------------------------------
-- 2. A physical temperature sensor (Temperature)
-----------------------------------------------------------------------------------------------
-- This is the name and idx of the REAL temperature sensor you want to use to monitor the
-- temperature. Virtual Temperature Sensor - name and id
-----------------------------------------------------------------------------------------------
-- Future improvement will be to define more than one temperature sensor and assume an average
-----------------------------------------------------------------------------------------------
--local currenttemperature = 'Temperature Kitchen'
--local currenttemperatureidx = 3
--local currenttemperature = 'Temperature Hall'
--local currenttemperatureidx = 111
local currenttemperature = 'Temperature Bedroom'
local currenttemperatureidx = 2
-----------------------------------------------------------------------------------------------
-- 3. A virtual switch to tun on and off the heating (On/Off Switch)
-----------------------------------------------------------------------------------------------
local virtualheater = 'Heating House'
local virtualheateridx = 178
-----------------------------------------------------------------------------------------------
-- 4. A virtual switch to set the target temperature (Temperature)
-----------------------------------------------------------------------------------------------
local targettemperature = 'Thermostat House'
local targettemperatureidx = 172
-----------------------------------------------------------------------------------------------
-- 5. A virtual switch to move the target temperature up (Push Button)
-----------------------------------------------------------------------------------------------
local remoteup = 'Thermostat House Up'
-----------------------------------------------------------------------------------------------
-- 6. A virtual switch to move the target temperature down (Push Button)
-----------------------------------------------------------------------------------------------
local remotedown = 'Thermostat House Down'
-----------------------------------------------------------------------------------------------
-- OPTIONS
-----------------------------------------------------------------------------------------------
-- Defines the amount the target temperature will move when the up and down buttons are pressed
-----------------------------------------------------------------------------------------------
local pitch= 1
-----------------------------------------------------------------------------------------------
-- The tolerance for the thermostat. So the heating doesn't continually turn on and off around
-- the target temperature this specifies the tolerance before any action is taken.
-----------------------------------------------------------------------------------------------
local tolerance = 0.5 -- NOT BEING USED!!!!!
-----------------------------------------------------------------------------------------------
-- After this time the heating will automatically turn off as a temperature was not detected
-- from the temperature sensor. this helps protect against the heating being left on all the
-- time if the signal is lost.
-----------------------------------------------------------------------------------------------
local lostsignaltime = 3600 -- 60 minutes
-----------------------------------------------------------------------------------------------
-- Helps protect against multiple quick changes to the physical switch
-----------------------------------------------------------------------------------------------
local heatercommanddelay = 30 -- 30 seconds
-----------------------------------------------------------------------------------------------
-- Repeats the On or Off command after a set time for devices that don't have a handshake
-- Should always be bigger than heatercommanddelay
-----------------------------------------------------------------------------------------------
local heaterrepeatdelay = 600 -- 1 minute
-----------------------------------------------------------------------------------------------
-- Set debug to 1 for testing. 0 for live running.
-----------------------------------------------------------------------------------------------
debug = 0 -- Stored as variable now
-----------------------------------------------------------------------------------------------
-----------------------------------------------------------------------------------------------
-----------------------------------------------------------------------------------------------
-----------------------------------------------------------------------------------------------
-----------------------------------------------------------------------------------------------
-- NOTHING SHOULD NEED TO CHANGE BELOW THIS LINE
-----------------------------------------------------------------------------------------------
-----------------------------------------------------------------------------------------------
-----------------------------------------------------------------------------------------------
-----------------------------------------------------------------------------------------------
-----------------------------------------------------------------------------------------------
local thermostatchgnagedetected = 0
commandArray = {}
function debuglog (s)
--if debug == 1 then print(s) end
--print "DEBUG"
if uservariables["Debug"] == 1 then print("DEBUG - THERMOSTATHOUSE " .. " " .. s) end
return true
end
function timedifference (s)
year = string.sub(s, 1, 4)
month = string.sub(s, 6, 7)
day = string.sub(s, 9, 10)
hour = string.sub(s, 12, 13)
minutes = string.sub(s, 15, 16)
seconds = string.sub(s, 18, 19)
t1 = os.time()
t2 = os.time{year=year, month=month, day=day, hour=hour, min=minutes, sec=seconds}
difference = os.difftime (t1, t2)
return difference
end
-----------------------------------------------------------------------------------------------
-- Actions for moving the target temperature up and down
-----------------------------------------------------------------------------------------------
if (devicechanged[remoteup] or devicechanged[remotedown]) then
target = tonumber(otherdevices_svalues[targettemperature])
debuglog('Thermostat - Target Temperature: ' .. tostring(target))
if (devicechanged[remoteup]=='On') then
commandArray['UpdateDevice'] = targettemperatureidx .. '|0|' .. tostring(target+pitch)
commandArray[remoteup] = 'Off'
debuglog('Thermostat - Target Temperature Up by : ' .. tostring(pitch) .. ' to : ' .. tostring(target+pitch))
elseif (devicechanged[remotedown]=='On') then
commandArray['UpdateDevice'] = targettemperatureidx .. '|0|' .. tostring(target-pitch)
commandArray[remotedown] = 'Off'
debuglog('Thermostat - Target Temperature Down by : ' .. tostring(pitch) .. ' to : ' .. tostring(target-pitch))
end
else
target = tonumber(otherdevices_svalues[targettemperature])
debuglog('Thermostat - Target Temperature: ' .. tostring(target) .. '')
end
-----------------------------------------------------------------------------------------------
-- Controls the heating based on the target and current temperatures
-----------------------------------------------------------------------------------------------
-- Is the heating scheduled to be on?
if (otherdevices[virtualheater]=='On') then
debuglog('Heating - Heating Is Scheduled To Be On: ' .. virtualheater )
-- what is the target temperature?
target = tonumber(otherdevices_svalues[targettemperature])
-- test to see if we can still see the thermostat. If not force the heating off
--debuglog('Heating - Control Switch: ' .. tostring(timedifference(otherdevices_lastupdate[currenttemperature])) .. ' LostSignalTime: ' .. tostring(lostsignaltime))
if ( timedifference(otherdevices_lastupdate[currenttemperature]) > lostsignaltime ) then
debuglog('Heating - WARNING: Last seen temperature sensor: '.. currenttemperature ..' ' .. tostring(otherdevices_lastupdate[currenttemperature]))
debuglog('Heating - WARNING: Signal Lost with temperature sensor for over '.. lostsignaltime ..' seconds: '.. currenttemperature .. ' (IDX: '.. currenttemperatureidx ..')')
debuglog('Heating - WARNING: forcing Heating OFF : '.. physicalheater)
-- commandArray['SendNotification']='Test' -- SEND NOTIFICATION
if( timedifference(otherdevices_lastupdate[physicalheater]) > heaterrepeatdelay) then -- checks to see if the command needs to be repeated
if (otherdevices[physicalheater]=='Off') then
debuglog('Heating - WARNING - Turning Physical Heater On: '.. physicalheater)
else
debuglog('Heating - WARNING - Turning Physical Heater On (Repeat Command): '.. physicalheater)
end
commandArray[physicalheater] = 'On'
else
debuglog('Heating - INFO: Not enough time has passed before issuing repeat command to switch: Only '.. timedifference(otherdevices_lastupdate[physicalheater]) .. ' seconds out of ' .. heaterrepeatdelay .. ' seconds')
end
else
debuglog('Heating - Last seen temperature sensor: '.. currenttemperature ..' ' .. tostring(otherdevices_lastupdate[currenttemperature]))
-- what is the current temperature?
--debuglog('STRING 1 Current - Return Value : ' .. tostring(otherdevices_svalues[currenttemperature]))
--debuglog('STRING 2 Current - Value Type : ' .. type(otherdevices_svalues[currenttemperature]))
--debuglog('STRING 3 Current - First Element : ' .. string.gmatch(otherdevices_svalues[currenttemperature], '([^;]+)')(1))
-- As some temperature devices also return other values as humidity this assumes the first value is the temperature in all cases
current = tonumber(string.gmatch(otherdevices_svalues[currenttemperature], '([^;]+)')(1))
debuglog('Heating - Target: ' .. tostring(target) .. ' Current: ' .. tostring(current))
if( timedifference(otherdevices_lastupdate[physicalheater]) > heatercommanddelay ) then -- checks to see if the heater has been turned off recently
debuglog('Heating - Enough time has passed since last change to switch: '.. timedifference(otherdevices_lastupdate[physicalheater]) .. ' seconds out of ' .. heatercommanddelay .. ' seconds')
if (current < target) then
debuglog('Heating - Turning Physical Heater On: '.. physicalheater)
if( timedifference(otherdevices_lastupdate[physicalheater]) > heaterrepeatdelay or devicechanged[remoteup] or devicechanged[remotedown] or devicechanged[virtualheater]) then -- checks to see if the command needs to be repeated
if (otherdevices[physicalheater]=='Off') then
debuglog('Heating - Turning Physical Heater On: '.. physicalheater)
else
debuglog('Heating - Turning Physical Heater On (Repeat Command): '.. physicalheater)
end
commandArray[physicalheater] = 'On'
else
debuglog('Heating - INFO: Not enough time has passed before issuing repeat command to switch: Only '.. timedifference(otherdevices_lastupdate[physicalheater]) .. ' seconds out of ' .. heaterrepeatdelay .. ' seconds')
end
else
debuglog('Heating - Turning Physical Heater Off: '.. physicalheater)
if( timedifference(otherdevices_lastupdate[physicalheater]) > heaterrepeatdelay or devicechanged[remoteup] or devicechanged[remotedown] or devicechanged[virtualheater]) then -- checks to see if the command needs to be repeated
if (otherdevices[physicalheater]=='On') then
debuglog('Heating - Turning Physical Heater Off: '.. physicalheater)
else
debuglog('Heating - Turning Physical Heater Off (Repeat Command): '.. physicalheater)
end
commandArray[physicalheater] = 'Off'
else
debuglog('Heating - INFO: Not enough time has passed before issuing repeat command to switch: Only '.. timedifference(otherdevices_lastupdate[physicalheater]) .. ' seconds out of ' .. heaterrepeatdelay .. ' seconds')
end
end
else
debuglog('Heating - INFO: Not enough time has passed before issuing command to switch: Only '.. timedifference(otherdevices_lastupdate[physicalheater]) .. ' seconds out of ' .. heatercommanddelay .. ' seconds')
end
end
else
debuglog('Heating - Turning Heating Off As Scheduled To Be Off')
if( timedifference(otherdevices_lastupdate[physicalheater]) > heatercommanddelay ) then -- NEED SOMETHING IN HERE TO STOP TURNING ON AND OFF CONSTANTLY
debuglog('Heating - Enough time has passed before issuing command to switch: '.. timedifference(otherdevices_lastupdate[physicalheater]) .. ' seconds out of ' .. heatercommanddelay .. ' seconds')
if( timedifference(otherdevices_lastupdate[physicalheater]) > heaterrepeatdelay or devicechanged[remoteup] or devicechanged[remotedown] or devicechanged[virtualheater]) then -- checks to see if the command needs to be repeated
if (otherdevices[physicalheater]=='On') then
debuglog('Heating - Turning Physical Heater Off: '.. physicalheater)
else
debuglog('Heating - Turning Physical Heater Off (Repeat Command): '.. physicalheater)
end
commandArray[physicalheater] = 'Off'
else
debuglog('Heating - INFO: Not enough time has passed before issuing repeat command to switch: Only '.. timedifference(otherdevices_lastupdate[physicalheater]) .. ' seconds out of ' .. heaterrepeatdelay .. ' seconds')
end
else
debuglog('Heating - INFO: Not enough time has passed before issuing command to switch: Only '.. timedifference(otherdevices_lastupdate[physicalheater]) .. ' seconds out of ' .. heatercommanddelay .. ' seconds')
end
end


This is the inside of the Homeeasy HE105 and what I hooked it up to on the Boiler (see the boiler manual for info on the other connections). So once I hooked this up I left the digistat that came with the boiler attached but just turned it all the way up to max so Domoticz can control the heating and I don't have to worry too much about connecting everything back up when I move out.
