Hi, @edje1205,
As I noticed that more users have difficulties to send data, received by e.g. MQTT or a HTTP request from Node Red to Domoticz, I have decided to make a kind of model. As not all weather stations present the same format and not all type of sensors are present the input may vary.
Domoticz supports the following weather sensors:
1. Temperature (will be presented under the Temperature tab).
2. Humidity (will be presented under the Temperature tab).
3. Barometer (will be presented under the Weather tab).
4. Combined sensors Temperature and Humidity (will be presented under the Temperature tab).
5. Combined sensors Temperature and Humidity and Barometer (will be presented under the Temperature tab AND under the Weather tab).
6. Combined sensors Temperature and Barometer (will be presented under the Temperature tab AND under the Weather tab).
7. Rain (will be presented under the Weather tab).
8. Wind (will be presented under the Weather tab).
9. Combined sensors Wind and Temperature and Chill (will be presented under the Temperature tab AND under the Weather tab).
10. UV (will be presented under the Weather tab).
11. Solar radiation (will be presented under the Weather tab).
As extra I have also included:
12. Text sensor (will be presented under the Utility tab).
13. Custom sensor (will be presented under the Utility tab).
Temperature tab
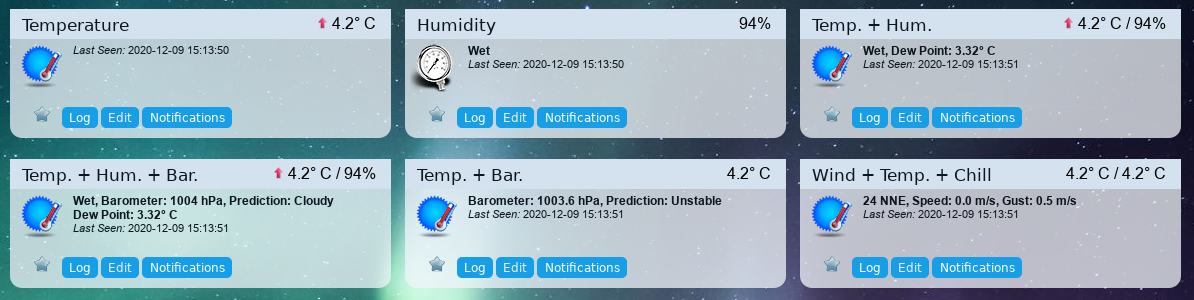
- Screenshot_weather_temp.png (243.49 KiB) Viewed 5136 times
Weather
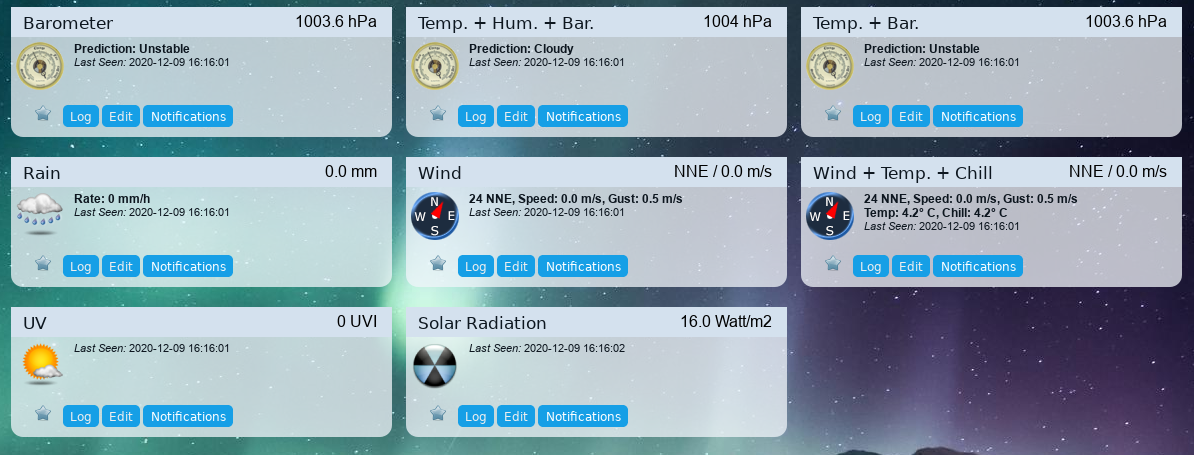
- Screenshot_weather_weather.png (367.86 KiB) Viewed 5136 times
Utility

- Screenshot_weather_utility.png (115.41 KiB) Viewed 5136 times
As an example we will use the following data, received from weatherstation Davis Vantage Pro 2.0 and weather program WeatherDisplay. (from developer Brian Hamilton)
Code: Select all
MQTTout : msg.payload : Object
object
ClientID: "Nieuwendam"
date: "15:29"
dateFormat: "d/m/y"
temp: 4.2
tempTL: 1.1
tempTH: 4.2
intemp: 17.6
dew: 3.3
dewpointTL: 0.5
dewpointTH: 3.4
apptemp: 3.2
apptempTL: -1.4
apptempTH: 4.8
wchill: 4.2
wchillTL: -2.4
heatindex: 4.2
heatindexTH: 4.2
humidex: 2.9
wlatest: 0
wspeed: 0
wgust: 0.5
wgustTM: 5.1
bearing: 24
avgbearing: 24
press: 1003.6
pressTL: 1000.2
pressTH: 1003.6
pressL: 812
pressH: 1046.9
rfall: 0
rrate: 0
rrateTM: 0
hum: 94
humTL: 94
humTH: 97
inhum: 48
SensorContactLost: "0"
forecast: "Meest bewolkt en koeler. Binnen 12 uur neerslag mogelijk, mogelijk met zware buien. Winderig."
tempunit: "C"
windunit: "m/s"
pressunit: "hPa"
rainunit: "mm"
temptrend: "+0.0"
TtempTL: "08:28"
TtempTH: "14:32"
TdewpointTL: "08:28"
TdewpointTH: "11:45"
TapptempTL: "00:41"
TapptempTH: "14:37"
TwchillTL: "14:32"
TheatindexTH: "14:32"
TrrateTM: "00:00"
ThourlyrainTH: ""
LastRainTipISO: "7/12/2020 15:14"
hourlyrainTH: 0
raintoday: 0
ThumTL: "13:13"
ThumTH: "09:12"
TpressTL: "00:00"
TpressTH: "15:24"
presstrendval: "+0.4"
Tbeaufort: 4
TwgustTM: "00:41"
windTM: 3.6
bearingTM: 186
timeUTC: "2020,12,08,14,29,52"
BearingRangeFrom10: 24
BearingRangeTo10: 24
UV: 0
UVTH: 0
SolarRad: 16
CurrentSolarMax: 43
SolarTM: 111
domwinddir: "Southeasterly"
windrun: 35.4
version: "10.37S"
build: "114"
ver: "10"
The flow consists of a simple MQTT input node. In this example the topic MQTTout is used.
The total payload is send to a "Function" node and from there to a MQTT output node.
This node has the topic domoticz/in.
The contents of the "Function" node is as follows:
Code: Select all
// This function sorts the comfort level for Domoticz based on the humidity.
function comfortlevel(hum) {
switch (true) {
case hum > 70: { hum_stat = 3 } break;
case hum < 30: { hum_stat = 2 } break;
case hum >= 30 && hum <= 45: { hum_stat = 0 } break;
case hum > 45 && hum <= 70: { hum_stat = 1 } break;
default: { hum_stat = 0 } break;
}
return hum_stat;
}
// This function sorts the prediction (barometer forecast) for Domoticz based on the barometer pressure.
function bar_forecast(pres) {
switch (true) {
case pres > 1030: { bar_for = 1 } break;
case pres > 1010 && pres <= 1030: { bar_for = 2 } break;
case pres > 990 && pres <= 1010: { bar_for = 3 } break;
case pres > 970 && pres <= 990: { bar_for = 4 } break;
default: { bar_for = 0 } break;
}
return bar_for;
}
// This function sorts the wind direction in abbreviated text for Domoticz based on the average bearing.
function winddir(avgb) {
switch (true) {
case avgb >= 0 && avgb <= 11.25: { dir = "N" } break;
case avgb >= 11.26 && avgb <= 33.75: { dir = "NNE" } break;
case avgb >= 33.76 && avgb <= 56.25: { dir = "NE" } break;
case avgb >= 56.26 && avgb <= 78.75: { dir = "ENE" } break;
case avgb >= 78.76 && avgb <= 101.25: { dir = "E" } break;
case avgb >= 101.26 && avgb <= 123.75: { dir = "ESE" } break;
case avgb >= 123.76 && avgb <= 146.25: { dir = "SE" } break;
case avgb >= 146.26 && avgb <= 168.75: { dir = "SSE" } break;
case avgb >= 168.76 && avgb <= 191.25: { dir = "S" } break;
case avgb >= 191.26 && avgb <= 213.75: { dir = "SSW" } break;
case avgb >= 213.76 && avgb <= 236.25: { dir = "SW" } break;
case avgb >= 236.26 && avgb <= 258.75: { dir = "WSW" } break;
case avgb >= 258.76 && avgb <= 281.25: { dir = "W" } break;
case avgb >= 281.26 && avgb <= 303.75: { dir = "WNW" } break;
case avgb >= 303.76 && avgb <= 326.25: { dir = "NW" } break;
case avgb >= 326.26 && avgb <= 348.75: { dir = "NNW" } break;
case avgb >= 348.76 && avgb <= 359.99: { dir = "N" } break;
default: { dir = "?" } break;
}
return dir;
}
var ba = msg.payload.press.toString(); //barometer pressure
var pr = bar_forecast(ba).toString(); // prediction (barometer forecast)
var tm = msg.payload.temp.toString(); // temperature
var hu = msg.payload.hum.toString(); // humidity
var lv = comfortlevel(hu).toString(); // comfort level
var rt = msg.payload.rrate.toString(); // rain rate
var fl = msg.payload.rfall.toString(); // rain fall
var sr = msg.payload.SolarRad.toString(); // solar radiation
var uv = msg.payload.UV.toString(); // UV radiation
var an = msg.payload.bearing.toString(); // wind bearing
var sp = (msg.payload.wspeed * 10).toString(); // wind speed in 0.1 m/s
var gu = (msg.payload.wgust * 10).toString(); // wind gust in 0.1 m/s
var ch = msg.payload.wchill.toString(); // chill temperature
var avgbr = msg.payload.avgbearing; // average bearing
var dr = winddir(avgbr); // wind direction in abbreviated text
var txt = msg.payload.forecast; // weather forecast
var dp = msg.payload.dew.toString(); // dew point
var msg1 = {};
var msg2 = {};
var msg3 = {};
var msg4 = {};
var msg5 = {};
var msg6 = {};
var msg7 = {};
var msg8 = {};
var msg9 = {};
var msg10 = {};
var msg11 = {};
var msg12 = {};
var msg13 = {};
msg1.payload = {"command":"udevice", "idx":30, "svalue":tm};
msg2.payload = {"command":"udevice", "idx":31, "nvalue":parseInt(hu), "svalue":lv};
msg3.payload = {"command":"udevice", "idx":32, "svalue":ba + ";" + pr};
msg4.payload = {"command":"udevice", "idx":33, "svalue":tm + ";" + hu + ";" + lv};
msg5.payload = {"command":"udevice", "idx":34, "svalue":tm + ";" + hu + ";" + lv + ";" + ba + ";" + pr};
msg6.payload = {"command":"udevice", "idx":35, "svalue":tm + ";" + ba + ";" + pr};
msg7.payload = {"command":"udevice", "idx":36, "svalue":rt + ";" + fl};
msg8.payload = {"command":"udevice", "idx":37, "svalue":an + ";" + dr + ";" + sp + ";" + gu + ";" + tm + ";" + ch};
msg9.payload = {"command":"udevice", "idx":38, "svalue":an + ";" + dr + ";" + sp + ";" + gu + ";" + tm + ";" + ch};
msg10.payload = {"command":"udevice", "idx":39, "svalue":uv + ";" + "0"};
msg11.payload = {"command":"udevice", "idx":40, "svalue":sr};
msg12.payload = {"command":"udevice", "idx":41, "svalue":txt};
msg13.payload = {"command":"udevice", "idx":42, "svalue":dp};
return [[msg1,msg2,msg3,msg4,msg5,msg6,msg7,msg8,msg9,msg10,msg11,msg12,msg13]];
The code consist of different parts.
1. As we need some parameters, which are not available in the received payload from the weather station we have to calculate these ourselves.
Therefore we will find 3 functions called comfortlevel, bar_forecast and winddir.
This functions are based on received parameters, like humidity for the comfort-level and bar (pressure) for the prediction (bar forecast).
The texts for the wind directions are based on the average bearing. If this is not available to current bearing must be used.
These texts can be changed to your local language. Do not use full text, but use abbreviations. (So NNE instead of Northnortheast)
These texts are to long and the sensor text does not fit nicely.
This part you will find from line 1 to 54.
2. As we want to send data to the different sensors, created in Domoticz we need some variables.
The majority of the variables are named after the variables used in:
https://piandmore.wordpress.com/2019/02 ... -domoticz/
These variables are introduced in order to avoid long, and there difficult to read, lines in the final command.
If variables are not available or not used, you can comment these out.09 Dec 2020, 16:57
This part you will find from line 56 to 72.
3. As we want to send more than 1 message from this "Function" node, we have declared 13 msg objects, called msg1 to msg13.
If you do not use some sensors, as they are not available or not preferred, you can comment these out.
This part you will find from line 74 to 86.
4. The different message objects are created, according to the syntax found at:
https://www.domoticz.com/wiki/Domoticz_ ... .2Fsensors
These syntax can not be changed, nor you can create your own sensors.
If you do not use some sensors, as they are not available or not preferred, you can comment these out.
This part you will find from line 88 to 100.
5. The output payload you will find at line 102.
If you do not use some sensors, as they are not available or not preferred, you should remove the corresponding msg* between the double square brackets [[ ]].
Do not forget to replace the Domoticz IDX numbers with your IDX numbers.
You will find these in lines 88-100 (indexes 30-42)
The complete Node Red flow, including 2 "Debug nodes"you will find below:
Code: Select all
[{"id":"a18ffc2.ff459","type":"debug","z":"47d75961.f88b6","name":"","active":true,"tosidebar":true,"console":false,"tostatus":false,"complete":"false","statusVal":"","statusType":"auto","x":550,"y":220,"wires":[]},{"id":"6d2265db.acae74","type":"mqtt in","z":"47d75961.f88b6","name":"MQTTout In","topic":"MQTTout","qos":"0","datatype":"json","broker":"f9f13036.e28b58","x":170,"y":260,"wires":[["f30d5e0e.6c1a58","e7b054b8.b89c2"]]},{"id":"f30d5e0e.6c1a58","type":"function","z":"47d75961.f88b6","name":"","func":"// This function sorts the comfort level for Domoticz based on the humidity.\n\nfunction comfortlevel(hum) {\n \nswitch (true) {\n case hum > 70: { hum_stat = 3 } break;\n case hum < 30: { hum_stat = 2 } break;\n case hum >= 30 && hum <= 45: { hum_stat = 0 } break;\n case hum > 45 && avgb <= 70: { hum_stat = 1 } break;\n default: { hum_stat = 0 } break;\n}\nreturn hum_stat;\n}\n\n// This function sorts the prediction (barometer forecast) for Domoticz based on the barometer pressure.\n\nfunction bar_forecast(pres) {\n\nswitch (true) {\n case pres > 1030: { bar_for = 1 } break;\n case pres > 1010 && pres <= 1030: { bar_for = 2 } break;\n case pres > 990 && pres <= 1010: { bar_for = 3 } break;\n case pres > 970 && avgb <= 990: { bar_for = 4 } break;\n default: { bar_for = 0 } break;\n}\nreturn bar_for;\n}\n\n// This function sorts the wind direction in abbreviated text for Domoticz based on the average bearing.\n\nfunction winddir(avgb) {\n\nswitch (true) {\n case avgb >= 0 && avgb <= 11.25: { dir = \"N\" } break;\n case avgb >= 11.26 && avgb <= 33.75: { dir = \"NNE\" } break;\n case avgb >= 33.76 && avgb <= 56.25: { dir = \"NE\" } break;\n case avgb >= 56.26 && avgb <= 78.75: { dir = \"ENE\" } break;\n case avgb >= 78.76 && avgb <= 101.25: { dir = \"E\" } break;\n case avgb >= 101.26 && avgb <= 123.75: { dir = \"ESE\" } break;\n case avgb >= 123.76 && avgb <= 146.25: { dir = \"SE\" } break;\n case avgb >= 146.26 && avgb <= 168.75: { dir = \"SSE\" } break;\n case avgb >= 168.76 && avgb <= 191.25: { dir = \"S\" } break;\n case avgb >= 191.26 && avgb <= 213.75: { dir = \"SSW\" } break;\n case avgb >= 213.76 && avgb <= 236.25: { dir = \"SW\" } break;\n case avgb >= 236.26 && avgb <= 258.75: { dir = \"WSW\" } break;\n case avgb >= 258.76 && avgb <= 281.25: { dir = \"W\" } break;\n case avgb >= 281.26 && avgb <= 303.75: { dir = \"WNW\" } break;\n case avgb >= 303.76 && avgb <= 326.25: { dir = \"NW\" } break;\n case avgb >= 326.26 && avgb <= 348.75: { dir = \"NNW\" } break;\n case avgb >= 348.76 && avgb <= 359.99: { dir = \"N\" } break;\n default: { dir = \"?\" } break;\n}\nreturn dir;\n}\n\nvar ba = msg.payload.press.toString(); //barometer pressure\nvar pr = bar_forecast(ba).toString(); // prediction (barometer forecast)\nvar tm = msg.payload.temp.toString(); // temperature\nvar hu = msg.payload.hum.toString(); // humidity\nvar lv = comfortlevel(hu).toString(); // comfort level\nvar rt = msg.payload.rrate.toString(); // rain rate\nvar fl = msg.payload.rfall.toString(); // rain fall\nvar sr = msg.payload.SolarRad.toString(); // solar radiation\nvar uv = msg.payload.UV.toString(); // UV radiation\nvar an = msg.payload.bearing.toString(); // wind bearing\nvar sp = (msg.payload.wspeed * 10).toString(); // wind speed in 0.1 m/s\nvar gu = (msg.payload.wgust * 10).toString(); // wind gust in 0.1 m/s\nvar ch = msg.payload.wchill.toString(); // chill temperature\nvar avgbr = msg.payload.avgbearing; // average bearing\nvar dr = winddir(avgbr); // wind direction in abbreviated text\nvar txt = msg.payload.forecast; // weather forecast\nvar dp = msg.payload.dew.toString(); // dew point\n\nvar msg1 = {};\nvar msg2 = {};\nvar msg3 = {};\nvar msg4 = {};\nvar msg5 = {};\nvar msg6 = {};\nvar msg7 = {};\nvar msg8 = {};\nvar msg9 = {};\nvar msg10 = {};\nvar msg11 = {};\nvar msg12 = {};\nvar msg13 = {};\n\nmsg1.payload = {\"command\":\"udevice\", \"idx\":30, \"svalue\":tm};\nmsg2.payload = {\"command\":\"udevice\", \"idx\":31, \"nvalue\":parseInt(hu), \"svalue\":lv};\nmsg3.payload = {\"command\":\"udevice\", \"idx\":32, \"svalue\":ba + \";\" + pr};\nmsg4.payload = {\"command\":\"udevice\", \"idx\":33, \"svalue\":tm + \";\" + hu + \";\" + lv};\nmsg5.payload = {\"command\":\"udevice\", \"idx\":34, \"svalue\":tm + \";\" + hu + \";\" + lv + \";\" + ba + \";\" + pr};\nmsg6.payload = {\"command\":\"udevice\", \"idx\":35, \"svalue\":tm + \";\" + ba + \";\" + pr};\nmsg7.payload = {\"command\":\"udevice\", \"idx\":36, \"svalue\":rt + \";\" + fl};\nmsg8.payload = {\"command\":\"udevice\", \"idx\":37, \"svalue\":an + \";\" + dr + \";\" + sp + \";\" + gu + \";\" + tm + \";\" + ch};\nmsg9.payload = {\"command\":\"udevice\", \"idx\":38, \"svalue\":an + \";\" + dr + \";\" + sp + \";\" + gu + \";\" + tm + \";\" + ch};\nmsg10.payload = {\"command\":\"udevice\", \"idx\":39, \"svalue\":uv + \";\" + \"0\"};\nmsg11.payload = {\"command\":\"udevice\", \"idx\":40, \"svalue\":sr};\nmsg12.payload = {\"command\":\"udevice\", \"idx\":41, \"svalue\":txt};\nmsg13.payload = {\"command\":\"udevice\", \"idx\":42, \"svalue\":dp};\n\nreturn [[msg1,msg2,msg3,msg4,msg5,msg6,msg7,msg8,msg9,msg10,msg11,msg12,msg13]];\n","outputs":1,"noerr":0,"initialize":"","finalize":"","x":360,"y":260,"wires":[["a18ffc2.ff459","c2547ec1.bd1aa"]]},{"id":"c2547ec1.bd1aa","type":"mqtt out","z":"47d75961.f88b6","name":"Domoticz In","topic":"domoticz/in","qos":"0","retain":"false","broker":"f9f13036.e28b58","x":550,"y":260,"wires":[]},{"id":"e7b054b8.b89c2","type":"debug","z":"47d75961.f88b6","name":"","active":true,"tosidebar":true,"console":false,"tostatus":false,"complete":"false","statusVal":"","statusType":"auto","x":370,"y":320,"wires":[]},{"id":"f9f13036.e28b58","type":"mqtt-broker","name":"localhost","broker":"127.0.0.1","port":"1883","clientid":"","usetls":false,"compatmode":true,"keepalive":"60","cleansession":true,"birthTopic":"","birthQos":"0","birthRetain":"false","birthPayload":"","closeTopic":"","closeQos":"0","closePayload":"","willTopic":"","willQos":"0","willPayload":""}]
Regards