I have a Bose Soundtouch 20 Series II (Airplay version), and I recently came across this python library
https://github.com/CharlesBlonde/libsoundtouch for accessing and controlling it.
I have created two simple (i.e., can be improved!) python scripts: selector and volume control.
Selector
This script uses a Domoticz selector switch to select from the 6 stored presets; I have extended it to add two streaming URLs, as well as the Soundtouch's AUX input:
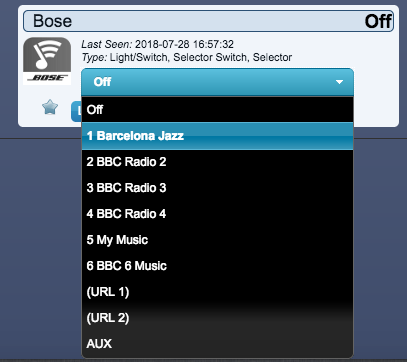
(I'm using 10 levels - 0 to 90 - but this can be extended / reduced as required.)
The code (see later) takes a single argument (0 to 9), corresponding to the above levels, and the selector actions have been set up as follows:
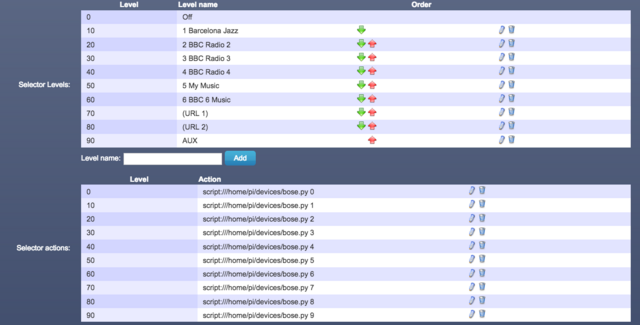
Obviously, change this to correspond to the names for your presets!
Code:
- Spoiler: show
Code: Select all
#!/usr/bin/env python
from libsoundtouch import soundtouch_device
from libsoundtouch.utils import Source, Type
import sys
bose = '<your Soundtouch wifi address>'
# put your stream URLs here
url1 = 'http://178.33.232.106:8034/stream'
url2 = 'http://direct.fipradio.fr/live/fip-webradio2.mp3'
device = soundtouch_device(bose)
device.power_on()
presets = device.presets()
# read arg
arg = int(sys.argv[1])
print arg
if arg == 0: # power off
device.power_off()
if 1 <= arg <= 6: # presets 1-6
device.select_preset(presets[arg-1])
if arg == 7: # HTTP URL (not HTTPS)
device.play_url(url1)
if arg == 8: # HTTP URL (not HTTPS)
device.play_url(url2)
if arg == 9: # AUX
device.select_source_aux()
Save this with the name and location specified in the selector actions (I used /home/pi/devices/bose.py).
Volume control
Set up a dummy switch in Domoticz, and change it to type 'dimmer' (0 - 100%).
This uses the following python script:
- Spoiler: show
Code: Select all
#!/usr/bin/env python
from libsoundtouch import soundtouch_device
from libsoundtouch.utils import Source, Type
from time import sleep
import requests
import json
bose = '<your Soundtouch wifi address>'
volIdx = '<Domoticz idx for dimmer switch>'
url = 'http://<domoticz url:port>/json.htm?type=devices&rid=' + volIdx
oldStatus = ''
device = soundtouch_device(bose)
device.power_on()
def domoticzread(var):
response = requests.get(url)
jsonData = json.loads(response.text)
result = jsonData['result'][0][var]
return result
while 1:
volume = domoticzread('Level')
status = domoticzread('Status')
if status != oldStatus:
#print volume, status
if status == 'Off':
device.set_volume(0)
else:
# status = 'On' or 'Set Level: <x> %'
device.set_volume(volume)
oldStatus = status
sleep(0.2)
This needs to be run in background, e.g.,
Code: Select all
python /home/pi/devices/bose_volume.py &
or started on boot in crontab.
(In both scripts, insert your own values in angle brackets: <...>)
I'm interested in any comments / suggestions for improvement.