Prepare Domoticz
If you don't know how to create a virtual sensor chances are you have never done this before.
- Go to "Hardware"
- Fill in the name field with a desired name (like "Virtual")
- Choose for type the "Dummy, does nothing, use for virtual switches only"
- Click on "Add"
- The added hardware is now added to the hardware list. In the same row there is a "Create virtual sensor" button shown. Click on it.
Hardware
Now you are ready to create virtual devices.
- Go to "Hardware"
- Press "Create virtual sensor".
- Fill in the name field with a name like 'Plex'
Devices
Now you are ready to create a virtual device.
- Go to "Hardware"
- Press "Create virtual sensor".
- Fill in the name field with a name like 'Plex'
Plex script
Config the parameters in the Plex script:
Code: Select all
#config plex url
plexURL = '<plex ip>:<plex port>' #base url (default port = 32400)
#domoticz settings
domoticz_host = ''
domoticz_port = ''
domoticz_url = 'json.htm'
#domoticz idx
domoticz_PlexStatus = '' #idx of device
Put the script under /domoticz/scripts and make the script executable:
"sudo chmod +x /home/pi/domoticz/scripts/plex.py"
Test
Run the script by calling:
"sudo python /home/pi/domoticz/scripts/plex.py"

If Plex Server is not playing anything, this is the result:
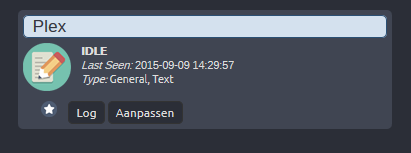
Create the Cronjob
Now all ingredients are available to read the data from the portal and insert them into Domoticz.
- execute "sudo crontab -e"
- add the following line "*/10 * * * * /home/pi/domoticz/scripts/plex.py"
- exit crontab. Now everything should be working for you.
Scripts
plex.py
Code: Select all
#!/usr/bin/python
import urllib, urllib2, hashlib
from xml.etree import ElementTree as ET
#config plex url
plexURL = '<plex ip>:<plex port>' #base url (default port = 32400)
#domoticz settings
domoticz_host = ''
domoticz_port = ''
domoticz_url = 'json.htm'
#domoticz idx
domoticz_PlexStatus = '' #idx of device
#building url
requestURL = 'http://'+plexURL+'/status/sessions'
print requestURL
try:
#call session url
test = urllib.urlopen(requestURL).read()
print test
root = ET.XML(test)
print root.tag
videotitle = root.find('Video').attrib['title']
print 'Video Playing: ' + videotitle
urllib.urlopen("http://" + domoticz_host + ":" + domoticz_port + "/" + domoticz_url+ "?type=command¶m=addlogmessage&message=Video Playing: " + videotitle)
#uploading values to domoticz
url = ("http://" + domoticz_host + ":" + domoticz_port + "/" + domoticz_url+ "?type=command¶m=udevice&idx=" + domoticz_PlexStatus + "&nvalue=0&svalue=Playing: " + videotitle)
urllib.urlopen(url)
except Exception:
print 'IDLE'
#uploading values to domoticz
url = ("http://" + domoticz_host + ":" + domoticz_port + "/" + domoticz_url+ "?type=command¶m=udevice&idx=" + domoticz_PlexStatus + "&nvalue=0&svalue=IDLE")
urllib.urlopen(url)
pass