I can confirm that this remote is working perfectly with Domoticz.
Also with some scripting I was able to get up to 24 (yes you read that correctly) different functions from the 8 buttons.
Eg I've got a "Radio button"
Single press = start playing radio, press it again = change to another track
Hold for more than 2 but less than 6 seconds = Stop playing
Hold for more than 6 seconds = add playing track to my like list
Can also do cool stuff with this remote using Lua scripting such as allocating one button as "Combo", eg press combo then light button = do x so you could make the light button do x if pressed individually or do something else if combo then light button pressed within 3 seconds.
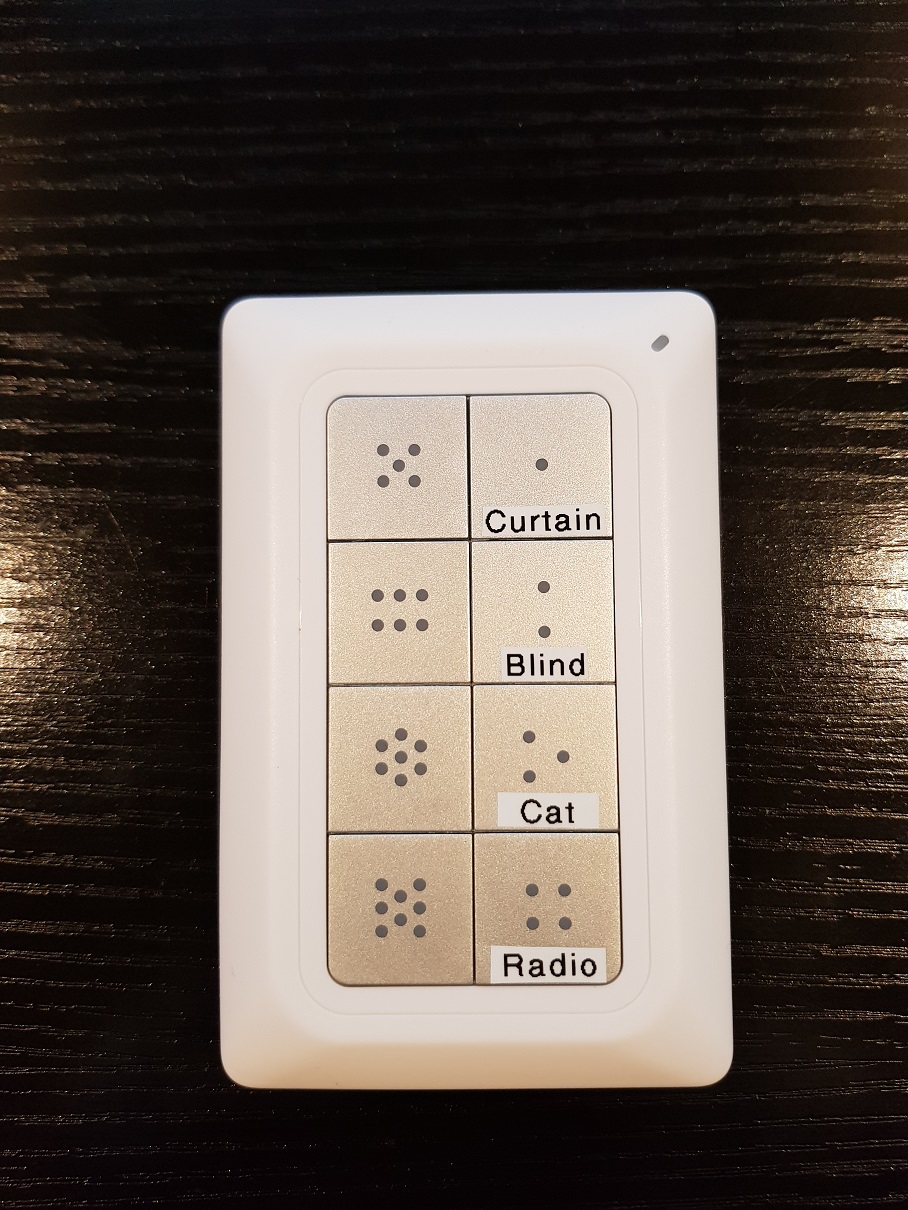
- 20180720_233329.jpg (362.9 KiB) Viewed 1366 times
PeterB1 start with this as a template Lua device script
Code: Select all
-- Device Last Updates
t1 = os.time()
devices = {
"Bedroom ZRC90 Curtain Button"
}
for i = 1, #devices do
s = otherdevices_lastupdate[devices[i]]
year = string.sub(s, 1, 4)
month = string.sub(s, 6, 7)
day = string.sub(s, 9, 10)
hour = string.sub(s, 12, 13)
minutes = string.sub(s, 15, 16)
seconds = string.sub(s, 18, 19)
t2 = os.time{year=year, month=month, day=day, hour=hour, min=minutes, sec=seconds}
str = (devices[i] .. "LastUpdate")
str = str:gsub("%s+", "")
str = string.gsub(str, "%s+", "")
_G[str] = (os.difftime (t1, t2))
end
commandArray = {}
-- Curtain button
if (devicechanged['Bedroom ZRC90 Curtain Button'] == 'On' and otherdevices['Main Bedroom Curtains'] == 'Closed' and BedroomZRC90CurtainButtonLastUpdate > 2) then
commandArray['Main Bedroom Curtains'] = 'On'
end
return commandArray
My ZRC90 Lua config
Code: Select all
-- Device Last Updates
t1 = os.time()
devices = {
"Bedroom ZRC90 Curtain Button",
"Bedroom ZRC90 Blind Button",
"Bedroom ZRC90 Cat Button",
"Bedroom ZRC90 Radio Button",
"Main Bedroom Blinds",
"Main Bedroom Curtains"
}
for i = 1, #devices do
s = otherdevices_lastupdate[devices[i]]
year = string.sub(s, 1, 4)
month = string.sub(s, 6, 7)
day = string.sub(s, 9, 10)
hour = string.sub(s, 12, 13)
minutes = string.sub(s, 15, 16)
seconds = string.sub(s, 18, 19)
t2 = os.time{year=year, month=month, day=day, hour=hour, min=minutes, sec=seconds}
str = (devices[i] .. "LastUpdate")
str = str:gsub("%s+", "")
str = string.gsub(str, "%s+", "")
_G[str] = (os.difftime (t1, t2))
end
commandArray = {}
-- Curtain button
if (devicechanged['Bedroom ZRC90 Curtain Button'] == 'On' and otherdevices['Main Bedroom Curtains'] == 'Closed' and BedroomZRC90CurtainButtonLastUpdate > 2) then
commandArray['Main Bedroom Curtains'] = 'On'
os.execute ('/root/scripts/tts/speak.sh "Main Bedroom R" "Opening curtain." "40" &')
elseif (devicechanged['Bedroom ZRC90 Curtain Button'] == 'On' and otherdevices['Main Bedroom Curtains'] == 'Open' and BedroomZRC90CurtainButtonLastUpdate > 2) then
commandArray['Main Bedroom Curtains'] = 'Off'
os.execute ('/root/scripts/tts/speak.sh "Main Bedroom R" "Closing curtain." "40" &')
end
-- Blind Button (order is important, blind stop must be at top)
if (devicechanged["Bedroom ZRC90 Blind Button"] == 'On' and otherdevices['Main Bedroom Blinds'] ~= 'Stopped' and otherdevices['Main Bedroom Blinds'] ~= 'Open' and MainBedroomBlindsLastUpdate < 30 and MainBedroomBlindsLastUpdate > 2) then
os.execute ('/root/scripts/tts/speak.sh "Main Bedroom R" "Blind stopped." "40" &')
commandArray['Main Bedroom Blinds'] = 'Stop'
elseif (devicechanged['Bedroom ZRC90 Blind Button'] == 'On' and otherdevices['Main Bedroom Blinds'] == 'Closed' and BedroomZRC90BlindButtonLastUpdate > 2) or
(devicechanged["Bedroom ZRC90 Blind Button"] == 'On' and otherdevices['Main Bedroom Blinds'] == 'Stopped' and MainBedroomBlindsLastUpdate > 2) then
commandArray['Main Bedroom Blinds'] = 'Off'
os.execute ('/root/scripts/tts/speak.sh "Main Bedroom R" "Raising the blind." "40" &')
elseif (devicechanged['Bedroom ZRC90 Blind Button'] == 'On' and otherdevices['Main Bedroom Blinds'] == 'Open' and BedroomZRC90BlindButtonLastUpdate > 2) then
commandArray['Main Bedroom Blinds'] = 'On'
os.execute ('/root/scripts/tts/speak.sh "Main Bedroom R" "Lowering the blind." "40" &')
end
-- Bedroom Cat Mode (Note to self: time taken for blinds to fully open or close is 29 seconds)
if (devicechanged['Bedroom ZRC90 Cat Button'] == 'On' and otherdevices['Main Bedroom Blinds'] == 'Open' and BedroomZRC90CatButtonLastUpdate > 2) then
os.execute ('/root/scripts/tts/speak.sh "Main Bedroom R" "Cat mode engaged." "40" &')
commandArray['Main Bedroom Curtains'] = 'Off'
commandArray['Main Bedroom Blinds'] = 'On FOR 19 SECONDS AFTER 1'
elseif (devicechanged['Bedroom ZRC90 Cat Button'] == 'On' and otherdevices['Main Bedroom Blinds'] == 'Closed' and BedroomZRC90CatButtonLastUpdate > 2) then
os.execute ('/root/scripts/tts/speak.sh "Main Bedroom R" "Cat mode engaged." "40" &')
commandArray['Main Bedroom Curtains'] = 'Off'
commandArray['Main Bedroom Blinds'] = 'Off FOR 10 SECONDS AFTER 1'
elseif (devicechanged['Bedroom ZRC90 Cat Button'] == 'On' and otherdevices['Main Bedroom Blinds'] == 'Stopped' and BedroomZRC90CatButtonLastUpdate > 2) then
os.execute ('/root/scripts/tts/speak.sh "Main Bedroom R" "Lowering the blind." "40" &')
commandArray['Main Bedroom Blinds'] = 'On'
commandArray['Main Bedroom Curtains'] = 'Off AFTER 1'
end
-- Radio Button
if (devicechanged['Bedroom ZRC90 Radio Button'] == 'On' and BedroomZRC90RadioButtonLastUpdate > 4) then
os.execute ('/root/scripts/zrc90/bedroom/radio.sh &')
end
return commandArray
Note that to I tell Lua to execute a bash script to achieve the functionality mentioned for the radio button.